Back to Basics: Software Language Essentials
January 24, 2024
Story
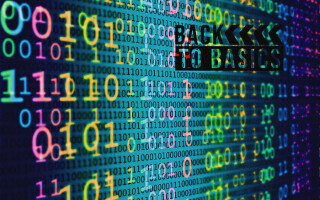
As I’m sure all of you know, software languages can be tricky to understand, especially when you consider the myriad of differences that separate each language from the others. But there are certain components that remain relatively consistent across most languages.
Welcome to Back to Basics, a series where we’re going to be reviewing basic engineering concepts that may require a more complex explanation than a quick Google search could provide.
We’re going to be explaining these concepts from the base level up to help you understand the basics of what software is all about.
At the most basic level, every computer is a machine that does stuff to numbers. It can add, subtract, multiply, and do a few other quirky things to numbers. But in order to do so effectively, it uses two important things: variables and operators.
Think of a variable as a box that holds numbers. Every variable has three important properties:
-
Address: This tells the computer where in its memory the variable “box” is located.
-
Size: This tells the computer how big of a number any given box can hold.
-
Contents: This is the actual number that will reside in the box.
A Matter of Size
An important point to make before we move on is that computers see the size of numbers differently than we do. When we see the number 5, we see it as one place value; likewise, a number like 243 obviously has three place values. These numbers work on the decimal system — that’s how we humans think about math.
But as you may know, computers only speak in zeroes and ones. When a computer looks at a number, it uses a different system called binary, which allows it to express the same number, but only using ones and zeroes. In binary, our examples from earlier (5 and 243), translate to 101 and 11110011, respectively.
So, when a computer creates a box, it looks at how many binary place values, or “bits,” that it needs to hold the number, rather than decimal place values — so a number like 11110011 takes eight bits of space in memory, while 101 takes three.
Now that we understand size a little better, we can look at the contents of a variable. We can pick any number we want to put in our variable “box” as long as the box can hold the corresponding number of bits. For example, an 8 bit variable has the capacity to hold the binary equivalent of any number up to 255.
An important thing to note is that once we pick the size of our box, it does not change. For consistency, let’s say we have an 8 bit variable. We then put the number 7 (binary 111) into our box. When we look at the box, we end up with 00000111. In order for the variable to stay the same size, it always will be 8 bits long. It still means the same thing, but it takes more place values. Fun, right? Not confusing at all. Let’s continue.
HE’S A SMOOTH OPERATOR
Once you have your variables established and their contents assigned, ideally, you’d want to do something with them.
Operators are the thing that the computer actually does to your variable. A computer is, at its most basic level, a fancy calculator. Variables allow the computer to move numbers around, but operators allow the computer to manipulate those variables.
Believe it or not, you actually can name most of the important operators used in code. If you look at the side of a calculator, you get a bunch of software operators: “+” (add), “-” (subtract), “*” (multiply), “/” (divide). Arithmetic operations make perfect sense for operators, since a computer is all about numbers.
If we think a little more, there are also a few other operators that also make sense: “<” (less than), “>” (greater than), “=” (equals). Comparison operators are great for knowing differences or similarities between variables.
Before we move on, there are a few operators that you may not have heard of: “&” (AND), “|” (OR), “<<” (bit shift left), “>>” (bit shift right). These are known as bitwise operators, and they allow the computer to manipulate binary numbers.
One of the easier ones to understand would be “&”, or AND. The AND operator takes two binary numbers, let’s say for example, 1011 & 0110. It then examines both numbers simultaneously, starting from the lowest place value and moving upward, and asks whether there is a 1 in each corresponding place value in the first AND second numbers. If the answer is yes, it puts a 1 in that place value of the result; if not, it puts a “0”. So, the solution to 1011 & 0110 = 0010. In the result, the only 1 is in the second place value, because it is in the second place value of both 1011 AND 0110.
Now that we have a good grasp of the two most fundamental parts of software, we are poised to move on to bigger fish! In our next article we’ll dig a little deeper into abstraction and syntax, but for now, congratulations on sticking with us on our journey into variables and operators!
This is part one of a series on Software Language Essentials, as part of our regular Back to Basics Feature. Click here for part two: Beyond Bytecode.
Click here for more Back to Basics.